C++/ C# introduction about each topics
COMPUTER SYSTEM:
A computer system is made of two major components: hardware and software. The computer hardware is the physical equipment. The software is the collection of programs (instructions) that allow the hardware to do its job.
Central processing unit
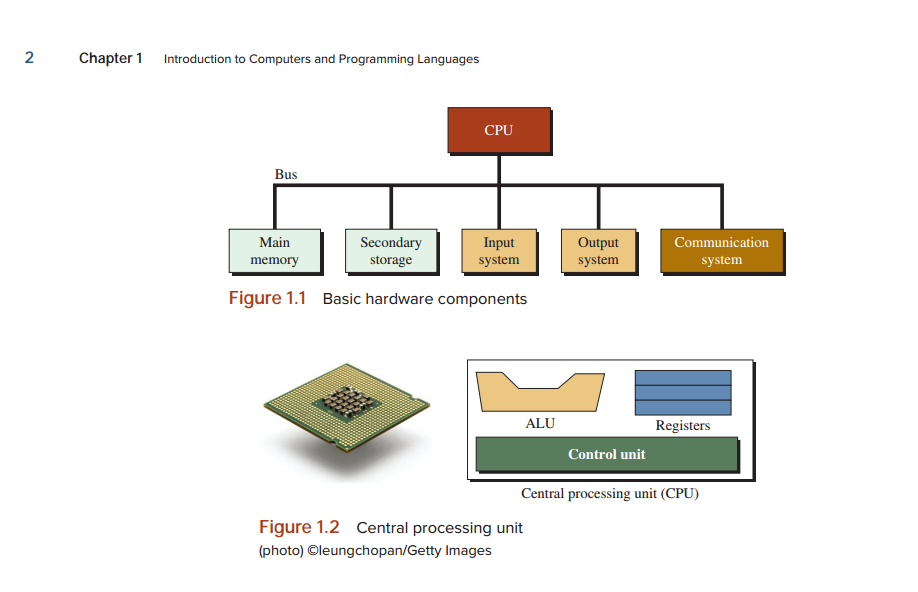
COMPUTER LANGUAGES
1 Machine Languages
2 Symbolic Languages
3 High-Level Languages: most notably BASIC, Pascal, Ada, C, C++, and Java—were developed.
Procedural Paradigm
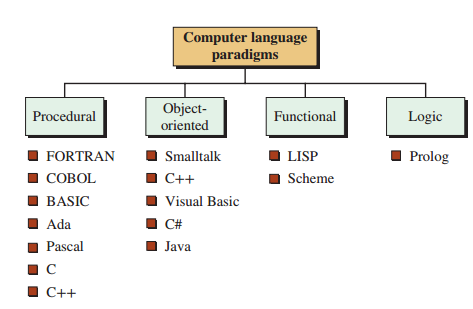
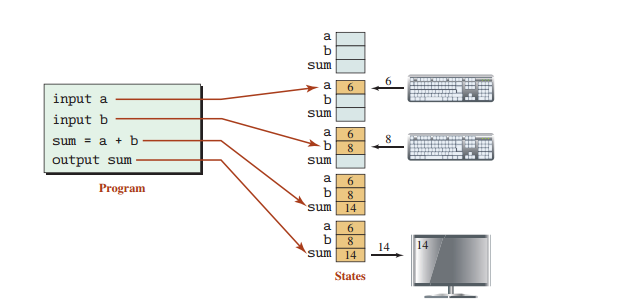
PROGRAM DESIGN
Find the largest number in a list of numbers
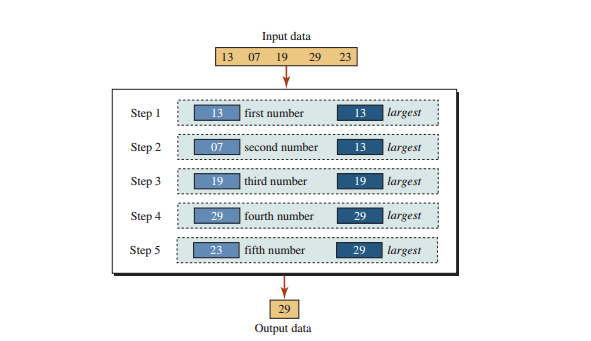
Algorithm Generalization
If the current number is greater than the largest, set the largest to the current number.
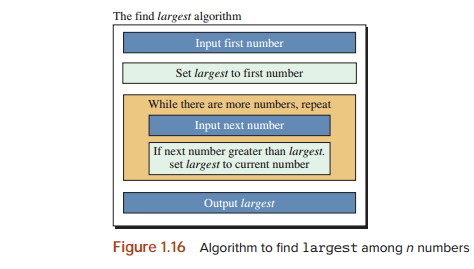
PROGRAM DEVELOPMENT
The procedure for a C++ program is a little bit more involved. The process is presented in a straightforward, linear fashion, but we need to recognize that these steps are repeated many times during the development process to correct errors and make improvements to the code.
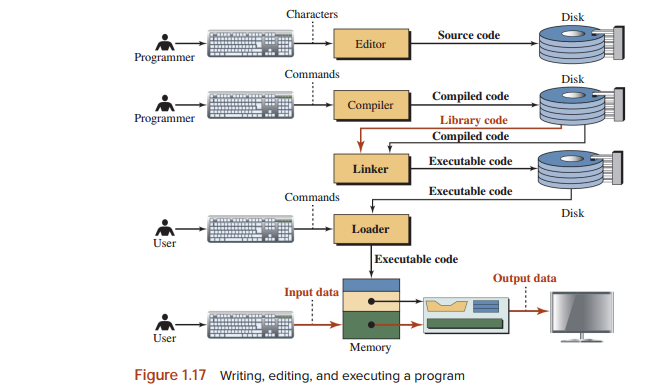
Basics of C++ Programming
Applications of C++:
- Operating Systems & Systems Programming. e.g. Linux-based OS (Ubuntu etc.)
- Browsers (Chrome & Firefox)
- Graphics & Game engines (Photoshop, Blender, Unreal-Engine)
- Database Engines (MySQL, MongoDB, Redis etc.)
- Cloud/Distributed Systems
we discussed machine language and high-level languages and showed a C11 statement. Because a computer can understand only machine language, you are ready to review the steps required to process a program written in C11.
Consider the following C11 program:
#include <iostream>
using namespace std;
int main()
{
cout << “My first C++ program.” << endl;
return 0;
}
Advantages of C++
- Portable language (writing a program irrespective of the operating system as well as Hardware)
- Low-level language like Assembly language on Machine language called portable.
- C++ use multi-paradigm programming. The Paradigm means the style of programming .paradigm concerned about logics, structure, and procedure of the program. C++ is multi-paradigm means it follows three paradigm Generic, Imperative, Object Oriented.
- It is useful for the low-level programming language and very efficient for general purpose.
- C++ provide performance and memory efficiency.
- It provides a high-level abstraction.
- In the language of the problem domain.
- C++ is compatible with C.
- C++ used reusability of code.
What is the difference between C & C++?
Why Use C++
- C++ is an object-oriented programming language that gives a clear structure to programs and allows code to be reused, lowering development costs.
- C++ is portable and can be used to develop applications that can be adapted to multiple platforms.
- As C++ is close to C# and Java it makes it easy for programmers to switch to C++ or vice versa.
C++ Install IDE
An IDE (Integrated Development Environment) is used to edit AND compile the code.
Popular IDE’s include Code::Blocks, Eclipse, and Visual Studio. These are all free, and they can be used to both edit and debug C++ code.
Note: Web-based IDE’s can work as well, but functionality is limited.
C++ Quickstart
Let’s create our first C++ file.
Open Codeblocks and go to File > New > Empty File.
In Codeblocks, it should look like this:
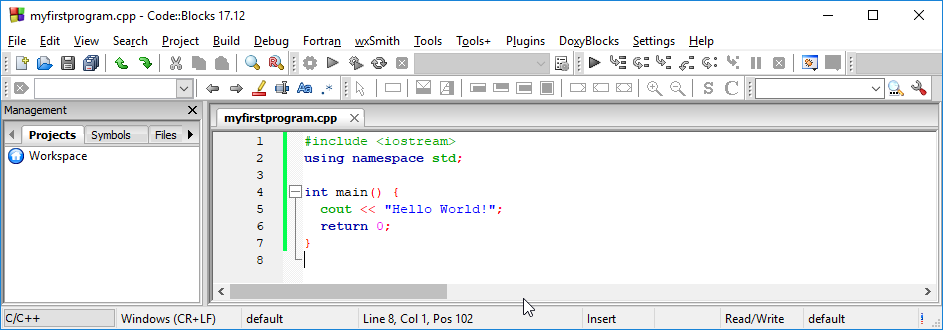
go to Build > Build and Run to run (execute) the program. The result will look something to this:
Hello World!
Process returned 0 (0x0) execution time : 0.011 s
Press any key to continue.
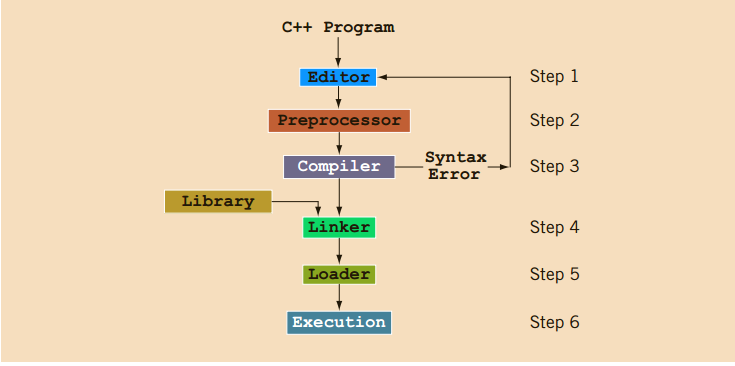
Problem analysis–coding–execution cycle
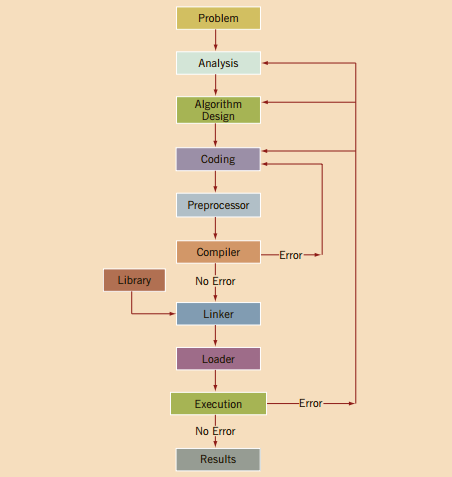
Algorithm Step | C11 Instruction (Code) |
1. Get the length of the rectangle. | cin >> length; |
2. Get the width of the rectangle. | cin >> width; |
3. Calculate the perimeter. | perimeter = 2 * (length + width); |
4. Calculate the area. | area = length * width; |
Program Analysis
#include <iostream>
The C++ designers created these lines of code and included them in files referred to as header files. We do not have to write these lines; we can simply copy the contents of these files into our code. To copy them, we need to know the name of the file, stream, which stands for input/
output stream in this case.
int main ()
Execution of each C++ program starts with the main function, which means that each program must have one function named main.
{
…
}
It is a major error to have an opening brace without a closing one or a closing brace without an opening one.
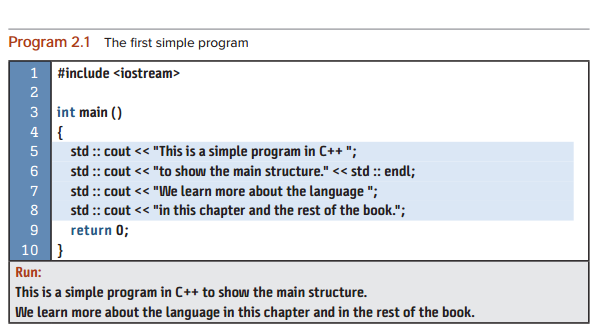
DATA TYPES
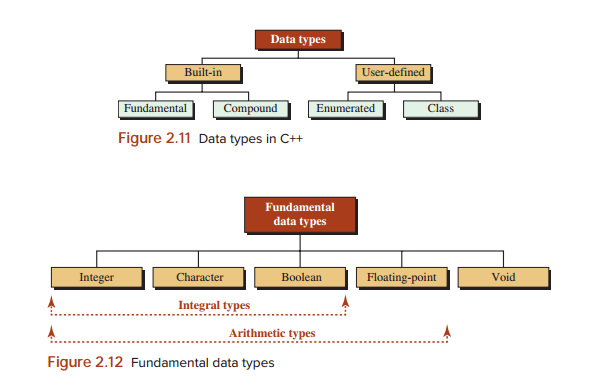
C++ How To Add Two Numbers
int main()
{
int x = 5;
int y = 6;
int sum = x + y;
cout << sum;
return 0;
}
Output -11
There are Six types of predefined identifiers |
include, iostream, main, std, cout, endl |
C# Tutorial
C# is a simple, modern, general-purpose, object-oriented programming language developed by Microsoft within its .NET initiative led by Anders Hejlsberg. This tutorial will teach you basic C# programming and will also take you through various advanced concepts related to C# programming language.
Notes: C# is pronounced as “C-Sharp”. It is an object-oriented programming language provided by Microsoft that runs on .Net Framework.
C# programming language, we can develop different types of secured and robust applications:
- Window applications
- Web applications
- Distributed applications
- Web service applications
- Database applications etc.
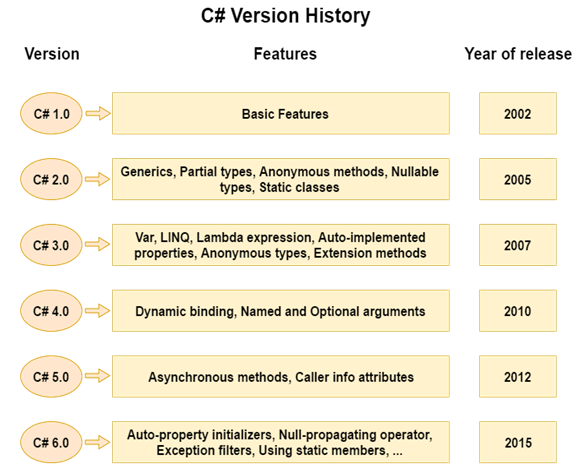
Java vs. C#
Java | C# |
Designed by Sun Microsystems | Designed as part of Microsoft’s .NET initiative. |
Java is a high-level, robust, secured, and object-oriented programming the language developed by Oracle. | C# is an object-oriented programming language developed by Microsoft that runs on .Net Framework. |
Invokes the “virtual” keyword in a base class and the “override” keyword in a derived class. | Enables polymorphism by default. |
Requires JDK to run Java | .Net framework provides a vast library of codes used by C# |
Eclipse, NetBeans, IntelliJ IDEA | Visual Studio, MonoDevelop |
Java doesn’t support the goto statement. | C# supports goto statement. |
Java supports JVM(Java Virtual Machine). | C# supports CLR(Common Language Runtime). |
Java does not support conditional compilation. | C# supports conditional compilation using preprocessor directives. |
Arrays in Java are a direct specialization of Object. | Arrays in C# are a specialization of the System. |
Java type safety is safe. | C# type safety is unsafe. |
C# Features
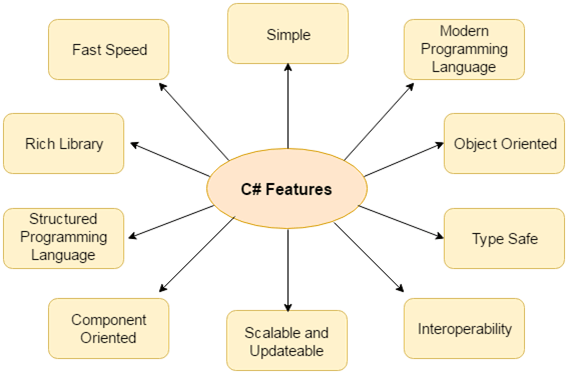
C# Data Types
Types | Data Types |
---|---|
Value Data Type | short, int, char, float, double, etc |
Reference Data Type | String, Class, Object, and Interface |
Pointer Data Type | Pointers |
C# operators
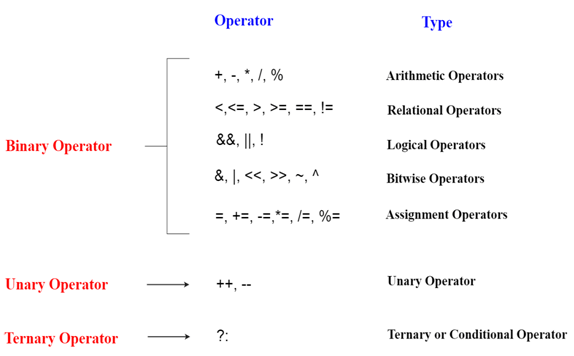
C# Keywords
abstract | base | as | bool | break | catch | case |
---|---|---|---|---|---|---|
byte | char | checked | class | const | continue | decimal |
private | protected | public | return | readonly | ref | sbyte |
explicit | extern | false | finally | fixed | float | for |
foreach | goto | if | implicit | in | in (generic modifier) | int |
ulong | ushort | unchecked | using | unsafe | virtual | void |
null | object | operator | out | out (generic modifier) | override | params |
default | delegate | do | double | else | enum | event |
sealed | short | sizeof | stackalloc | static | string | struct |
switch | this | throw | true | try | typeof | uint |
abstract | base | as | bool | break | catch | case |
volatile | while |
C# Goto Statement
The C# goto statement is also known jump statement. It is used to transfer control to the other part of the program. It unconditionally jumps to the specified label.
Example:
- {
- public static void Main(string[] args)
- {
- ineligible:
- Console.WriteLine(“You are not eligible to vote!”);
- Console.WriteLine(“Enter your age:\n”);
- int age = Convert.ToInt32(Console.ReadLine());
- if (age < 18){
- goto ineligible;
- }
- else
- {
- Console.WriteLine(“You are eligible to vote!”);
- }
- }
- }
Output:
You are not eligible to vote! Enter your age: 11 You are not eligible to vote! Enter your age: 5 You are not eligible to vote! Enter your age: 26 You are eligible to vote!
C# Variable
Variable Type | Example |
---|---|
Decimal types | decimal |
Boolean types | True or false value, as assigned |
Integral types | int, char, byte, short, long |
Floating-point types | float and double |
Basic Instruction
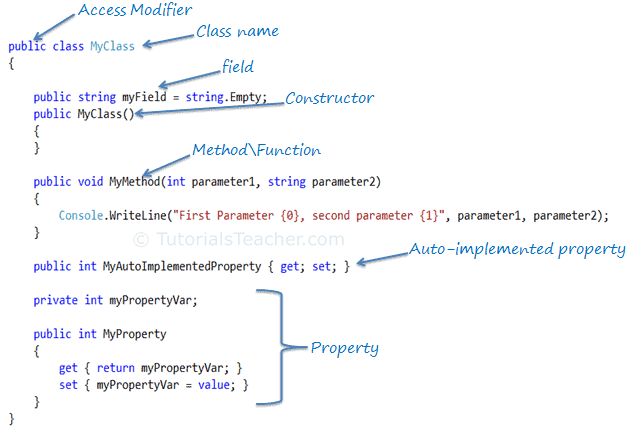
C# Methods
Different types of methods in C# programming,
- Parameter-less method
- Parameterized method
- Parameter and return method
- Call by value method
- Reference method
- Out parameter method
- Method overriding
- Method overloading
- Method hiding
- Abstract method
- Virtual method
- Static method
Book Pdf Download