PHP Introduction What is PHP?
- PHP: PHP Hypertext Preprocessor
- PHP is a scripting language for the Web that is used to develop Static websites or Dynamic websites or Web applications
- It is integrated with a number of popular databases, including MySQL, PostgreSQL, Oracle, Sybase, Informix, and Microsoft SQL Server.
- PHP is open Source software .
- PHP was created by Rasmus Lerdorf in 1995 but appeared in the market in 1995. PHP 7.4.0 is the latest version of PHP.
- PHP is faster than other scripting languages, for example, ASP and JSP.
- PHP can be embedded into HTML.
- It is powerful enough to be at the core of the biggest blogging system on the web (WordPress).
- It is also easy enough to be a beginner’s first server side language.
- PHP files can contain text, HTML, CSS, JavaScript, and PHP code.
Why PHP Use?
- PHP runs on various platforms (Windows, Linux, Unix, Mac OS X, etc.)
- PHP supports several protocols such as HTTP, POP3, SNMP, LDAP, IMAP, and many more.
- PHP is forgiving: PHP language tries to be as forgiving as possible.
- Two of the most famous examples of software written on PHP are Facebook and WordPress.
- One of the best things about PHP is the large Number of internet server providers (ISPs) and web hosting compaines that support it.
- PHP is free to easy to learn and runs efficiently on the server side.
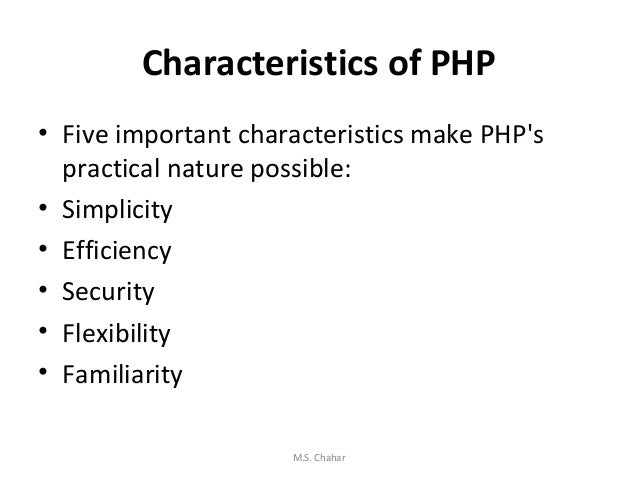

Prerequisite
HTML – HTML is used to design static webpage.
CSS – CSS helps to make the webpage content more effective and attractive.
JavaScript – JavaScript is used to design an interactive website.
Hello, World using PHP
<html> <head> <title>Hello World</title> </head> <body> <?php echo "Hello, World!";?> </body> </html>
Applications of PHP

- PHP can handle forms, i.e. gather data from files, save data to a file, through email you can send data, return data to the user.
- Web Content Management Systems
- GUI-Based Applications
- Using PHP, you can restrict users to access some pages of your website.
- The official PHP website (PHP.net) has installation instructions for PHP: http://php.net/manual/en/install.php
PHP Online Compiler / Editor
<?php
$txt = “PHP”;
echo “I love $txt!”;
?>
output: I love PHP!
Installing the required software
• PHP
• Nginx and Apache
• MongoDB
• MySQL
• GIT
• Redis
• Phalcon
PHP Variables
Rules for declaring PHP variable:
- A variable must start with a dollar ($) sign, followed by the variable name.
- A variable name must start with a letter or the underscore character.
- It can only contain alpha-numeric character and underscore (A-z, 0-9, _).
- PHP variables are case-sensitive, so $name and $NAME both are treated as different variable.
- A variable name cannot start with a number
PHP Variable: Declaring string, integer, and float,Booleans, NULL,Strings,Arrays,Objects,Resources
String | ?php $x = “Hello world!”; $y = ‘Hello world!’; echo $x; echo “<br>”; echo $y; ?> |
Integer | <?php $x = 5985; var_dump($x); ?> |
Float | <?php $x = 10.365; var_dump($x); ?> |
Boolean | $x = true; $y = false; |
Array | <?php $cars = array(“Volvo”,”BMW”,”Toyota”); var_dump($cars); ?> |
NULL Value | <?php $x = “Hello world!”; $x = null; var_dump($x); ?> |
PHP – Operator Types
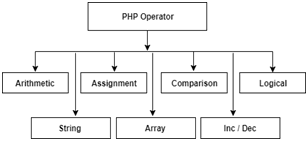
PHP Comments
A comment in PHP code is a line that is not executed as a part of the program. Its only purpose is to be read by someone who is looking at the code.
Single-Line Comments:
The single-line comment begins with “//” or “#”. When encountered, all text to the right will be ignored by the PHP interpreter.
// This is a comment
# This is also a comment
echo “Hello World!”; // This is also a comment, beginning where we see “//”
Multi-Line Comments: The multi-line comment can be used to comment out large blocks of code. It begins with /* and ends with */
/* This is a multi-line comment.
It spans multiple lines.
This is still part of the comment.
*/
PHP Functions
Variable-length argument lists | function variadic_func($nonVariadic, …$variadic) { echo json_encode($variadic); } variadic_func(1, 2, 3, 4); // prints [2,3,4] |
Optional Parameters | function hello($name, $style = ‘Formal’) { switch ($style) { case ‘Formal’: print “Good Day $name”; break; case ‘Informal’: print “Hi $name”; GoalKicker.com – PHP Notes for Professionals 123 break; case ‘Australian’: print “G’day $name”; break; default: print “Hello $name”; break; } } hello(‘Alice’); // Good Day Alice hello(‘Alice’, ‘Australian’); // G’day Alice |
Passing Arguments by Reference | function pluralize(&$word) { if (substr($word, -1) == ‘y’) { $word = substr($word, 0, -1) . ‘ies’; } else { $word .= ‘s’; } } |
Object arguments | function addOneDay($date) { $date->modify(‘+1 day’); } |
Basic Function Usage | function hello($name) { print “Hello $name”; } |
Variables inside functions | $number = 5 function foo(){ $number = 10 return $number } |
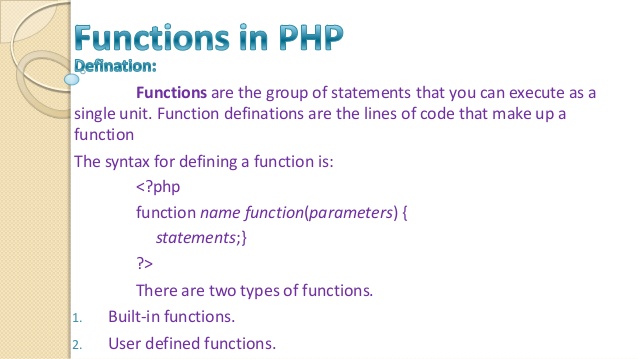
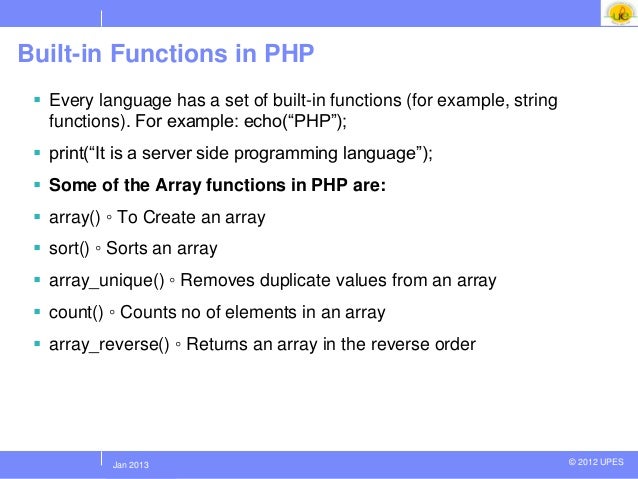
PHP v/s JavaScript
PHP | JavaScript |
Server-side Scripting language | Client-side Scripting language |
Used for back-end development | Mainly used for front-end development |
MariaDB,MySQL, and PostgreSQL | Combined with HTML,XML,Ajax |
It is synchronous by nature and waits for the I/O operation to execute. | JavaScript is asynchronous by nature and does not wait for I/O operation to execute. |
The Syntax is similar to C++ | the Syntax is similar to C |
Best for e-commerce and other website using CMS | MongoDB, CouchDB, and NoSQL |
PHP performs all the server-side functions like authentication, building custom web content, handling request, etc. | JavaScript is designed to create an interactive web application without interacting with the server |
In PHP, the code will be available and viewed after it is interpreted by the server | A JavaScript code can be viewed Even after the output is interpreted. |
Example-WordPress, Drupal, Joomla | Example-AngularJS and ReactJS: |
PHP vs HTML
PHP | HTML |
PHP is a server-side programming language. | HTML is a client-side scripting language. |
PHP is used in backend development, which interacts with databases to retrieve, store, and modify the information. | HTML is used in frontend development, which organizes the content of the website. |
PHP code executes on web servers like Apache web server, IIS web server. | HTML code executes on web browsers like Chrome, Internet Explorer, etc. |
PHP is a scripting language. | HTML is a markup language. |
PHP7.3 is the latest version of PHP. | HTML5.2 is the latest version of HTML. |

PHP File Upload
PHP file upload features allow you to upload binary and text files both. Moreover, you can have full control over the file to be uploaded through PHP authentication and file operation functions.
PHP File Upload Example: Code<form action=”uploader.php” method=”post” enctype=”multipart/form-data”>
Select File:
<input type=”file” name=”fileToUpload”/>
<input type=”submit” value=”Upload Image” name=”submit”/>
</form>
PHP Download File Example: Text File<?php
$file_url = ‘http://www.javatpoint.com/f.txt’;
header(‘Content-Type: application/octet-stream’);
header(“Content-Transfer-Encoding: utf-8”);
header(“Content-disposition: attachment; filename=\”” . basename($file_url) . “\””);
readfile($file_url);
?>
Types of Database Replication
Failover Replication: In this configuration, the replicated servers do not help with the load, but they do make sure that if the primary database
server goes down, there is a database with up-to-date information ready to take over.
Master/Replica Replication: In this configuration, the master database is the only one with reading/write access. The replica servers receive data as it is recorded on the master (or shortly thereafter)but are read-only copies of the master database. All updates go to the master database, but queries can go to either the master or any replica server. This is also known as “master/slave” replication, where the replica database is considered the “slave database.”
Multimaster Replication: In this configuration, all databases are considered to be equally “master” databases and writes can be performed on any of them. Writes to any given database are then synchronized with the rest of the cluster.
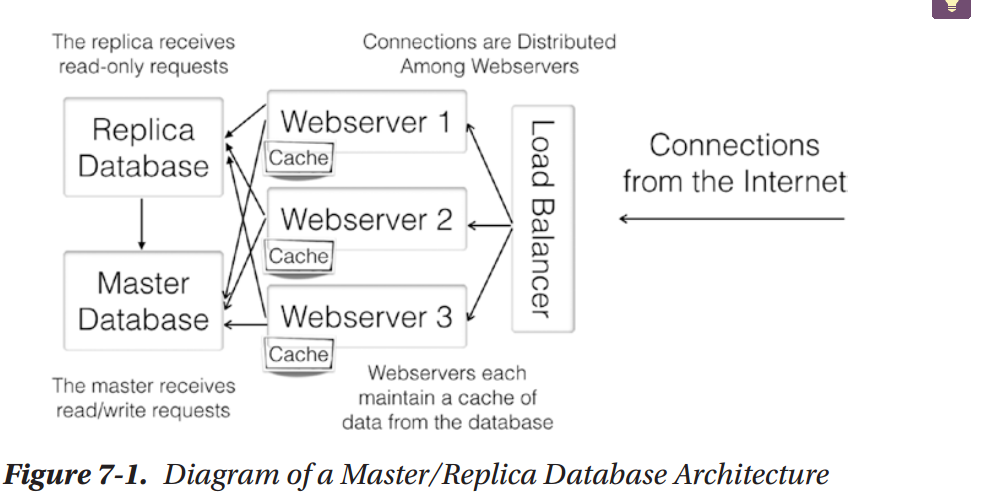
What is OOP?
Object-Oriented is an approach to software development that models applications around real-world objects such as employees, cars, bank accounts, etc. A class defines the properties and methods of a real-world object. An object is an occurrence of a class.
The Object-Oriented concepts in PHP are:
Class − This is a programmer-defined data type, which includes local functions as well as local data. You can think of a class as a template for making many instances of the same kind (or class) of the object.
Encapsulation: Reduce software development complexity – by hiding the implementation details and only exposing the operations, using a class becomes easy. Protect the internal state of an object – access to the class variables is via methods such as to get and set, this makes the class flexible and easy to maintain.
Polymorphism − This is an object-oriented concept where the same function can be used for different purposes. For example, the function name will remain the same but it makes take a different number of arguments and can do different tasks.
Inheritance: Re-usability– a number of children, can inherit from the same parent. This is very useful when we have to provide common functionality such as adding, updating, and deleting data from the database.
Methods Code
Static Methods | <?php class ClassName { public static function staticMethod() { echo “Hello World!”; } } ?> |
Static Properties | <?php class ClassName { public static $staticProp = “W3Schools”; } ?> |
LOOPS IN PHP | <?php echo “<p><b>Example of using the Break statement:</b></p>”; for ($i=0; $i<=10; $i++) { if ($i==3) { break; } echo “The number is “.$i; echo “<br />”; } ?> |
PHP Without a Web Server
Most PHP programmers have used PHP strictly in a web server environment. In such an environment, PHP is a CGI/Fast GGI or server module called and controlled by the HTTP server (usually Apache, IIS, Nginx, or similar). The HTTP server receives a request for a PHP-based web page and calls the PHP process to execute it, which usually returns output to the HTTP server to be sent on to the end-user.
There have been a number of attempts to create local applications (scripting systems, desktop apps, and so forth) with PHP using a locally installed web server, with some success.
For the value, they provide in these scenarios, web servers such as Apache are often over-specified and resource-hungry.
• Unless properly locked down, a web server running locally introduces a point of access to your machine for malicious visitors from the outside world.
• HTTP is a verbose protocol and (arguably) ideally suited for the Web. However, it’s often overkilled for local interprocess communication and adds more resource and complexity overhead to your application.
• Your application interface typically runs in a web browser and therefore looks anything but local (and comes with additional support/upgrade headaches unless you install a browser specifically for your app).
Book Pdf Download